When using PHPUnit, you'll want to either read the --help on the console, or refer to the online version, e.g. PHPUnit (v9.6) command line options as well as the rest of the documentation including the Appendix sections such as configuration.
If phpunit.xml or phpunit.xml.dist (in that order) exist in the current working directory and --configuration is not used, the configuration will be automatically read from that file.
The idea behind the fallback (to phpunit.xml.dist when phpunit.xml does not exist) is that phpunit.xml.dist can be checked into version control and phpunit.xml can be ignored from version control. This allows a developer to use a custom configuration without running the risk of accidentally checking it into version control. |
PHPUnit with VSCode[edit]
See PHPUnit/VSCode
For Semantic MediaWiki[edit]
First, refer to the Semantic MediaWiki website for info on Using PHPUnit
The notes below are my local additions or updates as I submit edits and Pull Requests to the canonical sources.
Test Suites[edit]
PHPUnit Test Suites are logical groups of files and/or directories that you can feed to PHPUnit for testing.
What are the test suites available to developers/testers in the SemanticMediaWiki codebase? The list-suites
option will tell us.
php ../../tests/phpunit/phpunit.php -c phpunit.xml.dist --list-suites
# or since that entrypoint is deprecated, we should call composer phpunit
# and pass our additional command line parameters after a --
composer phpunit -- -c phpunit.xml.dist --list-suites
- semantic-mediawiki-check - semantic-mediawiki-data-model - semantic-mediawiki-unit - semantic-mediawiki-integration - semantic-mediawiki-import - semantic-mediawiki-structure - semantic-mediawiki-benchmark
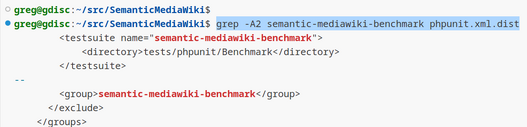
But where do those come from? What do they mean? What do they do? They are defined in phpunit.xml.dist
so you could inspect that file for more details.
For example, in that file, we can see that the "benchmark" test suite will process all the PHPUnit tests defined in the tests/phpunit/Benchmark
directory. (Aside if you didn't follow the link: you should execute the benchmark tests to compare master
against your feature
branch to catch performance regressions.)
To run the Semantic MediaWiki Unit test suite, and output a log in HTML format
php ../../tests/phpunit/phpunit.php -c phpunit.xml.dist --bootstrap tests/bootstrap.php --testsuite semantic-mediawiki-unit --testdox-html smw-unit-testdox.html
The text log is much more readable.
php ../../tests/phpunit/phpunit.php -c phpunit.xml.dist --bootstrap tests/bootstrap.php --testsuite semantic-mediawiki-unit --testdox-text smw-unit-testdox.txt
in a Docker Container[edit]
After you clone the code for SemanticMediaWiki and initialize the build submodule, you should be able to use make for various activities.
make bash
puts you into a bash shell as root in the wiki container
Change to the SMW directory if instead you are at the document root of /var/www/html
cd extensions/SemanticMediaWiki
root@2421517631d1:/var/www/html/extensions/SemanticMediaWiki#
Now you can manually interact with PHPUnit from the command line to accomplish precise objectives without having to wade through volumes of data and spend huge amounts of time waiting for entire test runs.
php ${MW_INSTALL_PATH:-../..}/tests/phpunit/phpunit.php -c phpunit.xml.dist --bootstrap tests/bootstrap.php --list-tests
lists the ~8800 tests such as:
SMW\Tests\Integration\SpecialsTest::testValidCovers
If you pipe it through grep, you can filter out the tests you want to run:
|grep JSONScript
such as
SMW\Tests\Integration\JSONScript\JSONScriptTestCaseRunnerTest::testCaseFile"a-0001.json"
But you can't simply pass these test names to the --filter
option of PHPUnit when trying to run the tests. This
php ../../tests/phpunit/phpunit.php -c phpunit.xml.dist --bootstrap tests/bootstrap.php --filter SMW\Tests\Integration\JSONScript\JSONScriptTestCaseRunnerTest::testCaseFile"a-0001.json"
only produces the result
No tests executed!
because the value is not delimited and wouldn't work "as is". The required format of the --filter parameter is defined as basically equivalent to the __METHOD__ magic constant. Also, the expected parameter value is a pattern, so if not enclosed in delimiters, the '/' delimiter will automatically be added. See the v9.6 docs
From the docs, valid --filter
parameter examples look like this:
--filter 'TestNamespace\\TestCaseClass::testMethod' --filter 'TestNamespace\\TestCaseClass' --filter TestNamespace --filter TestCaseClass --filter testMethod --filter '/::testMethod .*"my named data"/' --filter '/::testMethod .*#5$/' --filter '/::testMethod .*#(5|6|7)$/'
So, to properly invoke the above test, you could add single quotes and explicit delimiters with the name of the method plus use a wildcard in the pattern:
php ../../tests/phpunit/phpunit.php -c phpunit.xml.dist --bootstrap tests/bootstrap.php --filter '/::testCaseFile .*0001.json/'
or broaden the scope with a "pattern" that matches the class name - in which case all such tests would run (about 300).
php ../../tests/phpunit/phpunit.php -c phpunit.xml.dist --bootstrap tests/bootstrap.php --filter JSONScriptTestCaseRunnerTest
This form - using single quotes, with explicit pattern delimiters and just a short string - matches the same set of tests in our case.
php ../../tests/phpunit/phpunit.php -c phpunit.xml.dist --bootstrap tests/bootstrap.php --filter '/JSONScript/'
Results:
Tests: 338, Assertions: 5818, Failures: 12, Skipped: 21, Risky: 2.